Wikipedia define factorial as following
In mathematics, the factorial of a non-negative integer n, denoted by n!, is the product of all positive integers less than or equal to n.
Math is Fun gives the following definition
The factorial function (symbol: !) means to multiply a series of descending natural numbers.
Example
4! = 4 × 3 × 2 × 1 = 24
7! = 7 × 6 × 5 × 4 × 3 × 2 × 1 = 5040
1! = 1
0! = 1, because the following reason.
We can rearrange one thing only one way, as 0 is also an entity so we can rearrange it in one way.
Also we can prove it mathematically with the help of Combination
Let us consider n is the number of different people, if we choose a team consisting with n people then we can only choose it one time, so
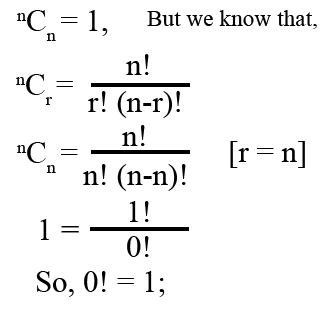
Now lets start coding using for loop
#include<stdio.h> int main(){ int n,i; float fact=1; printf("Enter your n\n"); scanf("%d",&n); if(n <0 ) printf("\aPlease enter a positive number\n"); else if((n == 1) || (n == 0)){ printf("\aThe factorial is 1\n"); } else{ for(i=1;i<=n;i++){ fact =fact*i; } printf("\aThe factorial is %.2f\n",fact); } return 0; }
Using while loop
#include<stdio.h> int main(){ int n,i=1; float fact=1; printf("Enter your n\n"); scanf("%d",&n); if(n <0 ) printf("\aPlease enter a positive number\n"); else if((n == 1) || (n == 0)){ printf("\aThe factorial is 1\n"); } else{ while(i<=n){ fact =fact*i; i++; } printf("\aThe factorial is %.2f\n",fact); } return 0; }
Using user defined function
#include<stdio.h> float factorial( int n){ if((n == 1) || (n == 0) ) return 1; else return n*factorial(n-1); } int main(){ int n; printf("Enter your n\n"); scanf("%d",&n); if(n <0 ) printf("\aPlease enter a positive number\n"); else printf("\aThe factorial is %.2f\n",factorial(n)); return 0; }