Row-major order and Column-major order: In computing, row-major order and column-major order describe methods for arranging multidimensional arrays in linear storage such as memory. The difference is simply that in row-major order, consecutive elements of the rows of the array are contiguous in memory; in column-major order, consecutive elements of the columns are contiguous.(Wiki)
C Supports Row-major order: That means if we represents a two dimensional matrix in C, At first It will traverse the first row then second row and so on.
Square Matrix: We know that square matrix is a matrix whose ROW and COLUMN are equal. If a square matrix of size n then ROW = COLUMN = n. Now see a square matrix. On which we will manipulate the main diagonal elements, elements above main diagonal, elements bellow the main diagonal.
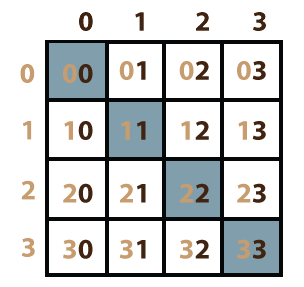
From the above image we can see that the indexes of all the diagonal elements follow a general rule, that is ROW = COLUMN, here [00], [11], [22] and [33].
Above the main diagonal we have [01], [02], [03], [12], [13] and [23]. Now notice carefully that, all these indexes follows ROW < COLUMN.Now we have those elements which are bellow the main diagonal these are, [10], [20], [21], [30], [31], and [32]. in these case ROW > COLUMN. Now lets start with code……
#include <stdio.h> int main(){ int mat[10][10], array_size, row=0, col=0, diagonalSum=0, aboveSum=0, bellowSum=0; printf("Enter the array size\n"); scanf("%d",&array_size); printf("Enter the elements of matrix\n"); for(row=0;row<array_size;row++){ for(col = 0;col<array_size;col++){ printf("Enter the element at [%d%d] : ",row,col); scanf("%d",&mat[row][col]); } printf("\n"); } // Sum of diagonal element ROW = COLUMN printf("The diagonal elements are \t"); for(row=0;row<array_size;row++){ for(col = 0;col<array_size;col++){ if(row==col){ printf(" %d, ",mat[row][col]); diagonalSum += mat[row][col]; } } } printf("\nAnd the sum is %d\n\n",diagonalSum); // Sum of elements above diagonal ROW < COLUMN printf("The elements above diagonal are \t"); for(row=0;row<array_size;row++){ for(col = 0;col<array_size;col++){ if(row<col){ printf(" %d, ",mat[row][col]); aboveSum += mat[row][col]; } } } printf("\nAnd the sum is %d\n\n",aboveSum); // Sum of elements bellow diagonal ROW > COLUMN printf("The elements bellow diagonal \t"); for(row=0;row<array_size;row++){ for(col = 0;col<array_size;col++){ if(row>col){ printf(" %d, ",mat[row][col]); bellowSum += mat[row][col]; } } } printf("\nAnd the sum is %d\n\n",bellowSum); return 0; }